With a custom keyboard, you can provide your application’s users with a personalized and improved experience. Customize the layout and appearance of the keyboard to add additional functionality and reflect your brand identity.
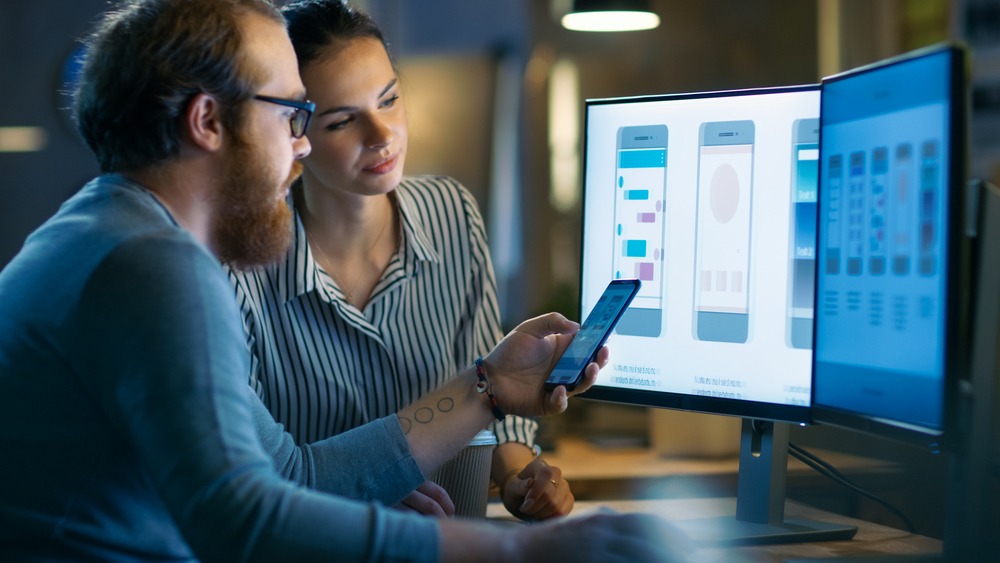
In this article, we will tell you how you can create a custom keyboard in android applications. But if you can afford it, you should hire Android developers to create custom keyboards instead. Such developers are very affordable on an hourly basis.
They can help you build a high-quality keyboard that meets the specific needs of your app. They will design, develop, test, and maintain your Android application and keyboard.
Although we have outlined the 9 steps in great detail, android developers have the programming skills and experience to build a high-quality keyboard that meets the diverse needs of the users.
Hire Android developers who are familiar with the Android platform. They can ensure that the keyboard is optimized for use on Android devices. Additionally, hiring an Android developer allows you to focus on other aspects of your business while the developer works on creating the custom keyboard.
Nine Steps to Build a Custom Keyboard:
Step 1: Create a new project on Android Studio.
To open Android Studio:
- Visit the Android Studio website (https://developer.android.com/studio). Click the Download Android Studio button (in green below).
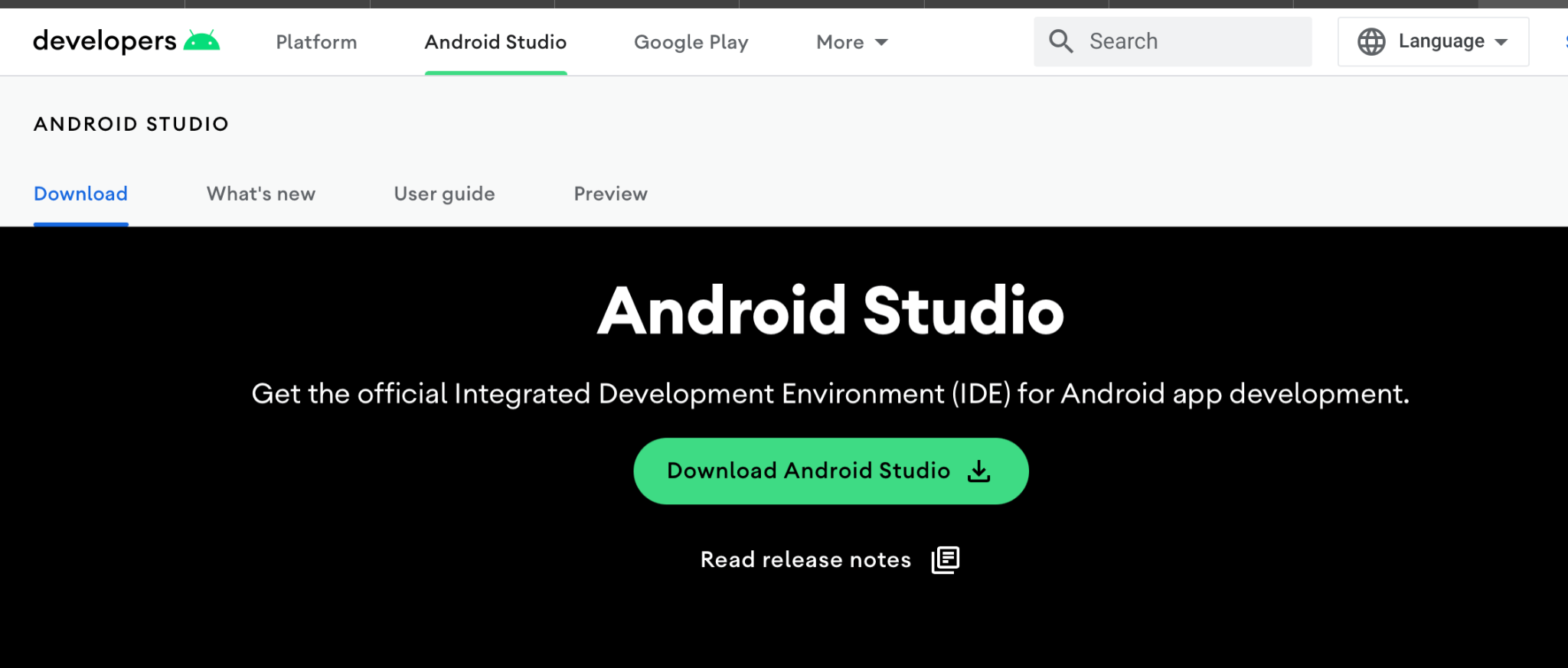
- Double-click the downloaded .dmg file to open it.
- Drag the Android Studio icon into the Applications folder.
- Double-click the icon in the Applications folder to open Android studio.
Alternatively, you can open Android Studio from the command line by using the open command:
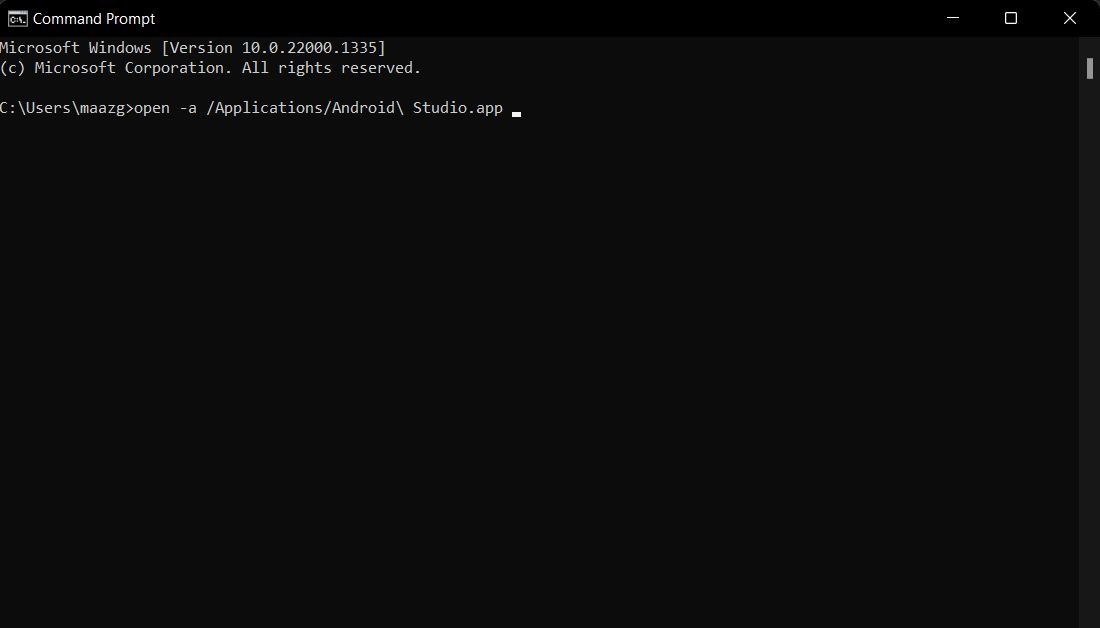
Copy the following command:
open -a /Applications/Android\ Studio.app
Step 2: In the project, make a new package inside the src folder. Name it inputmethod.
- Right-click on the src folder in the project tree on the left side of the Android Studio window.
- Select New from the menu that appears.
- Select Package from the submenu that appears.
- In the New Package dialog that appears, enter a name for the package in the Name field. A package is a directory that contains your source code files. Note – you should group related classes in the same package.
- Click the OK button to create the package.
Step 3: Make a new class that extends the InputMethodService class, inside the inputmethod package. Call it MyKeyboard.
- Right-click on the inputmethod package in the project tree on the left side of the Android Studio window.
- Select New from the menu that appears.
- Select Java Class from the submenu that appears.
- In the New Java Class dialog that appears, enter a name for the class in the Name field. This will be the name of your custom keyboard class.
- Select the InputMethodService class from the Superclass dropdown menu. This will make your custom keyboard class inherit the methods and functionality of the InputMethodService class.
- Click the OK button to create the class.
Step 4: Override the onCreateInputView() method in the MyKeyboard class. You can use this to set up the layout and buttons for the keyboard.
Use the following code:
@Override
public View onCreateInputView() {
// Inflate the keyboard layout
LayoutInflater inflater = (LayoutInflater) getSystemService(Context.LAYOUT_INFLATER_SERVICE);
View view = inflater.inflate(R.layout.keyboard, null);
// Set up the keyboard buttons
Button buttonA = (Button) view.findViewById(R.id.buttonA);
buttonA.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// Insert the character 'A' into the edit field
getCurrentInputConnection().commitText("A", 1);
}
});
// Repeat the process for other keyboard buttons
return view;
}
Step 5: In the res folder, Create a new directory called xml.
- Right-click on the res folder in the project tree on the left side of the Android Studio window.
- Select New from the menu that appears.
- Select the Directory from the submenu that appears.
- In the New Directory dialog that appears, enter xml in the Directory name field. This will create a new directory called xml inside the res folder.
- Click the OK button to create the directory.
Step 6: Inside the XML directory, make a new file called keyboard.xml. This file will define the appearance of the keyboard.
- Right-click on the XML directory in the project tree on the left side of the Android Studio window.
- Select New from the menu that appears.
- Select XML Resource File from the submenu that appears.
- In the New XML Resource File dialog that appears, enter the keyboard in the File name field. This will create a new XML file called keyboard.xml inside the XML directory.
- Click the OK button to create the file.
Step 7: In the keyboard.xml file, set up the layout for the keyboard. This can include buttons, text fields, and other stuff.
Use this code:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<Button
android:id="@+id/buttonA"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="A" />
<Button
android:id="@+id/buttonB"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="B" />
<!-- Add other buttons here -->
</LinearLayout>
<EditText
android:id="@+id/inputField"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</LinearLayout>
Step 8: In the AndroidManifest.xml file, add the android:name attribute to the <service> element to specify the name of the MyKeyboard class.
- Open the AndroidManifest.xml file in the app directory of your project.
- Find the <service> element and add the android:name attribute to it, like this: <service android:name=”.inputmethod.MyKeyboard”> </service>
- This will tell Android to use your MyKeyboard class as the service for the custom keyboard.
Step 9: Run the app and try out the custom keyboard.
- Click the Run button in the toolbar to build and run the app.
- In the device emulator that appears, open an app that allows you to enter text, such as a messaging app or a text editor.
- Tap the text input field to bring up the keyboard.
- Tap the Settings button (the gear icon) in the keyboard to open the keyboard settings.
- In the keyboard settings, tap the Input Method option and select your custom keyboard from the list of available keyboards.
- Tap the Done button to close the keyboard settings and return to the app.
Congratulations! Your custom keyboard is now active.
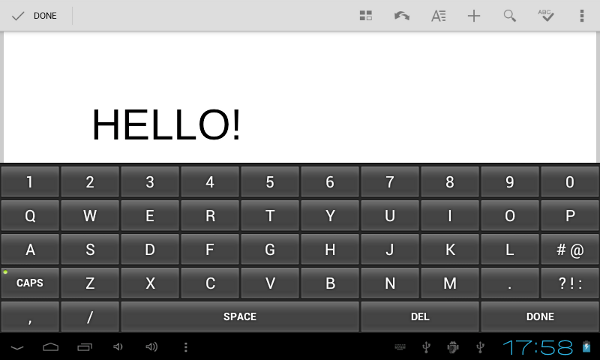
Certain aspects of making such a keyboard is outside the scope of one article. It requires experience and proper training, as we cannot predict specific needs. We have provided you with only the general process.
We recommend that you hire android developers to help you:
- Design the layout and appearance of the keyboard using XML and Java code.
- Implement the functionality of the keyboard buttons using Java code.
- Test the keyboard to ensure that it works correctly and is user-friendly.
- Maintain and update the keyboard as needed, such as fixing bugs or adding new features.
Hire Android developers to create a successful and user-friendly custom keyboard for your Android app while you focus on more important things for your organization.
Author Bio:
Justin D’Costa is an SEO specialist who works with Uplers, India’s largest hiring platform that helps remote-first companies to hire top remote tech talent.
Justin is an absolute digital marketing geek having an experience of more than half a decade. He has vast knowledge in the field of SEO, PPC, Web Analytics, and Web Development that he likes to share through excellent articles.
When not wrapping his head around marketing, you can find him playing with his dog, Casper, or enjoying the beautiful nature by spending some time on the beach.