A popular JavaScript library for creating user interfaces is React. How to invoke a child method from a parent component is one of the difficulties experienced by React developers. We’ll discuss a variety of approaches to develop this functionality with React in this post.
Before we examine the remedy, let’s examine the problem in more detail. Parts of a React application serve as the program’s framework.
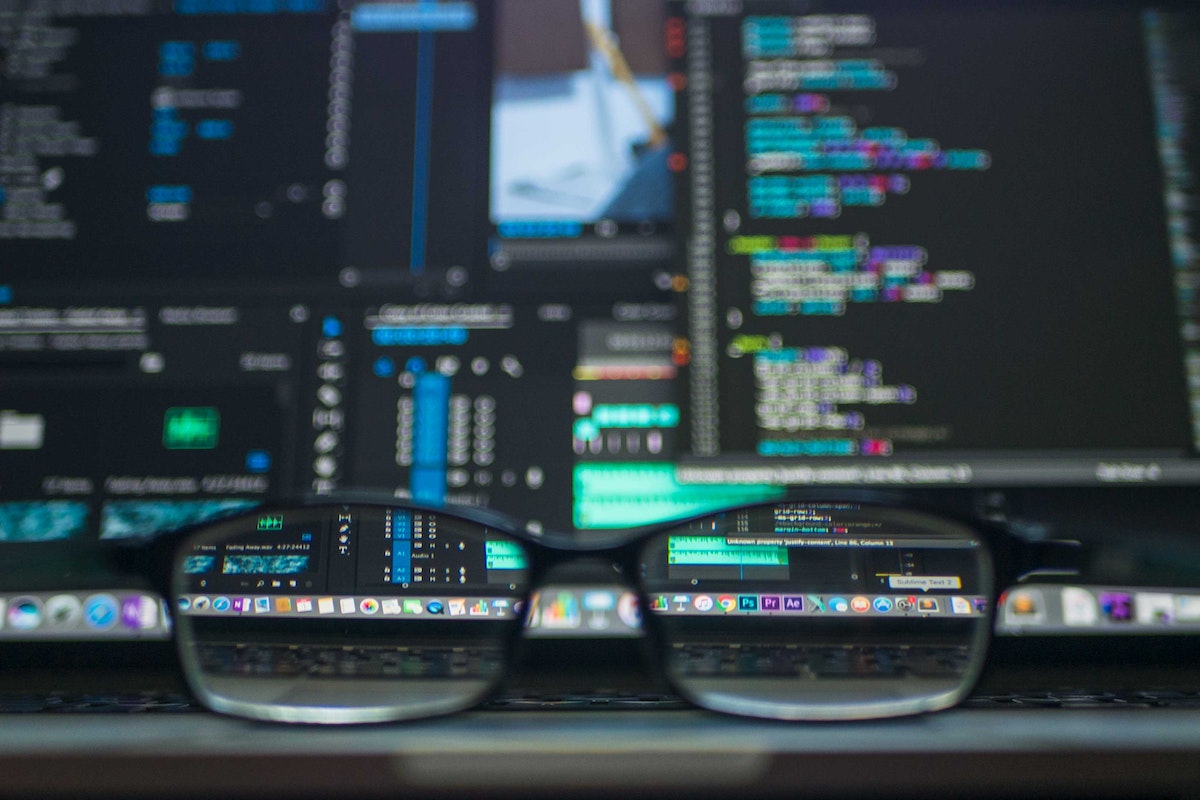
Each component has its own state and set of operations. Sometimes, a parent component must call a child method. A certain method on the child component should be called when a user clicks a button on the parent component.
React provides the following methods for calling child methods from parent components:
Making Use of Callbacks and Props:
Props and callbacks are two techniques for invoking a child method from a parent component. The parent component gives the child component a callback function as a prop. Callback functions allow child components to communicate with their parents.
Here is an example:
// Parent component
import React from 'react';
import ChildComponent from './ChildComponent';
class ParentComponent extends React.Component {
handleClick = () => {
this.child.handleClickFromParent();
}
render() {
return (
<div>
<ChildComponent handleClickFromParent={(child) => this.child = child} />
<button onClick={this.handleClick}>Call Child Method</button>
</div>
);
}
}
export default ParentComponent;
// Child component
import React from 'react';
class ChildComponent extends React.Component {
handleClickFromParent = () => {
console.log('Child method called from parent!');
}
render() {
return (
<div>
<h1>Child Component</h1>
</div>
);
}
}
export default ChildComponent;
The parent component provides the child component with a prop containing the handleClickFromParent callback function. The child component stores a duplicate of this callback function in its local state. The handleClick method is called when a user clicks a button on the parent component.
Refs:
A child function can also be called from a parent component using React refs. Child components are referenced when their methods are called by their parents.
Here is an example:
// Parent component
import React from 'react';
import ChildComponent from './ChildComponent';
class ParentComponent extends React.Component {
handleClick = () => {
this.child.handleClickFromParent();
}
render() {
return (
<div>
<ChildComponent ref={(child) => this.child = child} />
<button onClick={this.handleClick}>Call Child Method</button>
</div>
);
}
}
export default ParentComponent;
// Child component
import React from 'react';
class ChildComponent extends React.Component {
handleClickFromParent = () => {
console.log('Child method called from parent!');
}
render() {
return (
<div>
<h1>Child Component</h1>
</div>
);
}
}
export default ChildComponent;
The ref property creates a reference between the parent and child components. When a child component is clicked on by the user, the handleClick function invokes the handleClickFromParent method using the ref.
Contexts:
You can also call child functions from a parent component using context in React. You can move data down the component tree using context instead of manually entering props at every level.
Here is an example:
// Parent component
import React from 'react';
import ChildComponent from './ChildComponent';
export const ChildContext = React.createContext();
class ParentComponent extends React.Component {
handleClick = () => {
this.context.handleClickFromParent();
}
render() {
return (
<div>
<ChildContext.Provider value={{ handleClickFromParent: this.handleClick }}>
<ChildComponent />
</ChildContext.Provider>
<button onClick={this.handleClick}>Call Child Method</button>
</div>
);
}
}
ParentComponent.contextType = ChildContext;
export default ParentComponent;
// Child component
import React from 'react';
import { ChildContext } from './ParentComponent';
class ChildComponent extends React.Component {
static contextType = ChildContext;
handleClick = () => {
this.context.handleClickFromParent();
}
render() {
return (
<div>
<h1>Child Component</h1>
<button onClick={this.handleClick}>Call Parent Method</button>
</div>
);
}
}
export default ChildComponent;
In this example, the parent component creates a context using React.createContext(). The handleClick method is added to the context value, and the context is passed down to the child component using the ChildContext.Provider component. The child component uses the ChildContext.Consumer component to access the handleClickFromParent method from the context value.
To conclude, there are various ways to call a child method from a parent component in React, including props and callbacks, refs, and contexts. Each of these strategies has benefits and drawbacks, and the developer’s choice ultimately chooses which strategy to use.
However, references and context may result in a close relationship between parent and child components, which lowers the maintainability and reuse of the code. When feasible, it is best to utilize props and callbacks rather than refs and context. It is essential to hire a React JS developer with expertise with these methods.
A developer can help your business produce high-quality React apps with more features and flexibility.